Mouse interaction with meshes in matlab
Alec Jacobson
December 30, 2010
Here's a small piece of code illustrating how to receive mouse clicks on a mesh plotted with trisurf in matlab. Assuming your mesh is stored with vertices in V and face indices in F, then have onmeshdown
be the function you want to be called when the mesh is clicked and onvoiddown
be the function you want to be called when the void in the plot outside the mesh is clicked (the white and the grey parts).
tsh = trisurf(F,V(:,1),V(:,2),V(:,3),'tag','trisurf','ButtonDownFcn',@onmeshdown);
p = get(tsh,'Parent');
set(p,'ButtonDownFcn',@onvoiddown);
Here's a little demo showing you what you can do with that:
function dragdemo(V,F)
figure
tsh = trisurf(F,V(:,1),V(:,2),V(:,3),'ButtonDownFcn',@onmeshdown);
p = get(tsh,'Parent');
set(p,'ButtonDownFcn',@onvoiddown);
view(2);
axis equal
axis manual
down_pos = [];
drag_pos = [];
down_V = [];
function onvoiddown(src,ev)
% do nothing
end
function onmeshdown(src,ev)
down_pos=get(gca,'currentpoint');
title(sprintf('(%1.0f,%1.0f)',down_pos(1,1,1),down_pos(1,2,1)));
set(gcf,'windowbuttonmotionfcn',@drag)
set(gcf,'windowbuttonupfcn',@up)
down_V = get(tsh,'Vertices');
end
function drag(src,ev)
drag_pos=get(gca,'currentpoint');
title( ...
sprintf( ...
'(%1.0f,%1.0f) -> (%1.0f,%1.0f)', ...
down_pos(1,1,1),down_pos(1,2,1), ...
drag_pos(1,1,1),drag_pos(1,2,1)));
set( ...
tsh, ...
'Vertices', ...
[ ...
(drag_pos(1,1,1) - down_pos(1,1,1)) + down_V(:,1), ...
(drag_pos(1,2,1) - down_pos(1,2,1)) + down_V(:,2), ...
down_V(:,3) ...
]);
end
function up(src,ev)
set(gcf,'windowbuttonmotionfcn','');
set(gcf,'windowbuttonupfcn','');
end
end
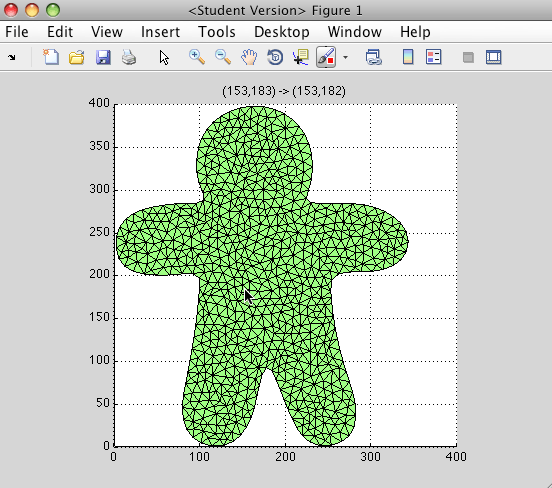